🎈前言
这篇文章男女通用,看懂了就去给异性讲吧。
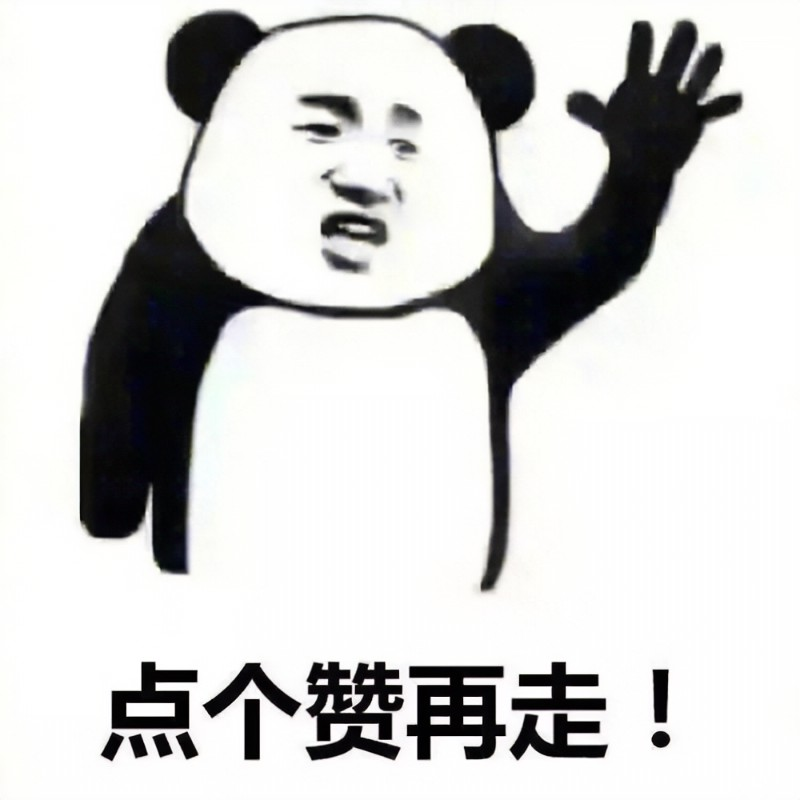
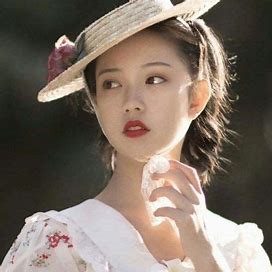
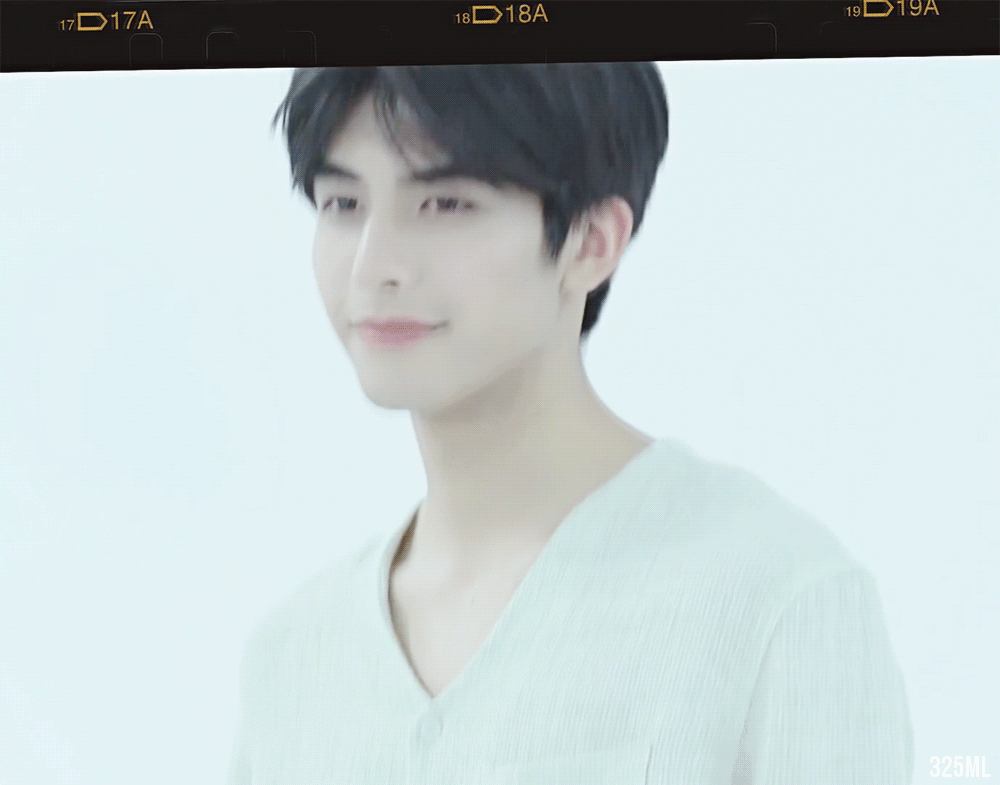
🎈什么是栈
可以把栈当成是弹夹,只能从栈顶放入数据和取出数据,而且每次取出的时候都是从栈顶取,先进后出后进先出
🎈动图演示栈
🎈链表实现栈:
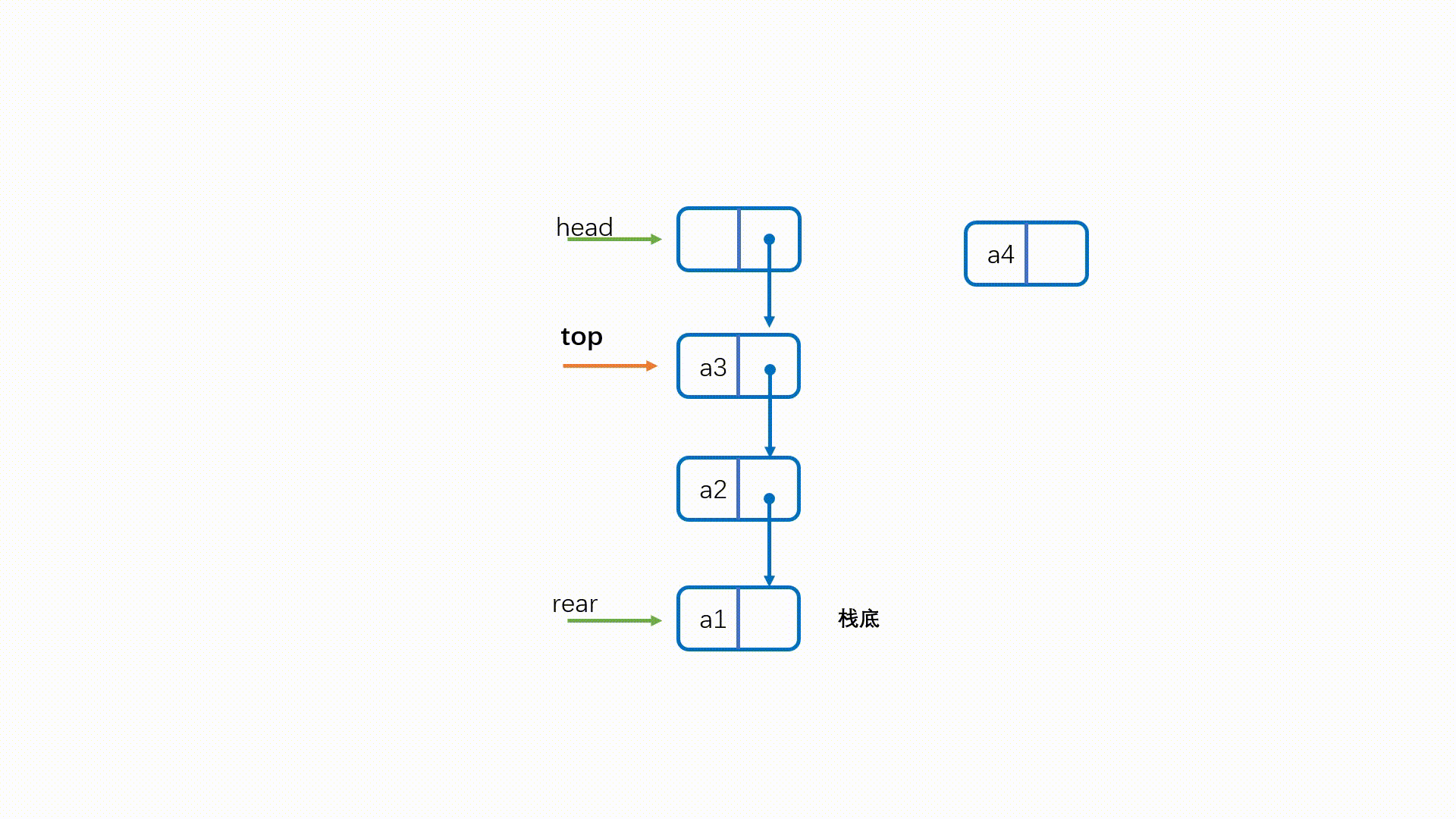
🎈线性表实现栈:
🎈栈的具体实现
🎈stak.h 定义函数,结构体,所需要的头文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| #pragma once #include<stdio.h> #include<stdlib.h> #include<assert.h> #include<stdbool.h> typedef int STDataType;
typedef struct Stack { STDataType* a; int top; int capacity; } ST; void StackInit(ST*ps); void StackPush(ST* ps, STDataType x); void StackPop(ST*ps); int StackSize(ST*ps); bool StackEmpty(ST* ps); STDataType StackTop(ST* ps); void Destroy(ST* ps);
|
🎈stack.c实现函数
🎈 StackInit(ST*ps);//初始化栈
1 2 3 4 5 6 7 8
| void StackInit(ST* ps) { assert(ps); ps->a = NULL; ps->top = 0; ps->capacity = 0; }
|
🎈StackPush(ST* ps, STDataType x);//入栈
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| void StackPush(ST* ps, STDataType x) { assert(ps); if (ps->top == ps->capacity) { int newCapacity = ps->capacity == 0 ? 4 : (ps->capacity * 2); ps->a = (STDataType*)realloc(ps->a, sizeof(STDataType) * newCapacity); if (ps->a == NULL) { printf("realloc fail\n"); exit(-1); } ps->capacity = newCapacity; }
ps->a[ps->top] = x; ps->top++; }
|
🎈StackPop(ST*ps);//出栈
1 2 3 4 5 6 7
| void StackPop(ST* ps) { assert(ps); assert(ps->top > 0); --ps->top; }
|
🎈StackSize(ST*ps);//栈中数据的数量
1 2 3 4 5 6
| int StackSize(ST* ps) { assert(ps); return ps->top; }
|
🎈StackEmpty(ST* ps);//判断栈是否为空
1 2 3 4 5 6 7
| bool StackEmpty(ST* ps) { assert(ps);
return (ps->top == 0); }
|
🎈StackTop(ST* ps);//栈顶元素
1 2 3 4 5 6 7 8
| STDataType StackTop(ST* ps) { assert(ps); assert(ps->top > 0); return ps->a[ps->top - 1]; }
|
🎈Destroy(ST* ps);//销毁栈
1 2 3 4 5 6 7
| void Destroy(ST* ps) { assert(ps); free(ps->a); ps->top = ps->capacity = 0; }
|
🎈stack.c完整代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| #define _CRT_SECURE_NO_WARNINGS 1 #include"stack.h" void StackInit(ST* ps) { assert(ps); ps->a = NULL; ps->top = 0; ps->capacity = 0; }
void StackPush(ST* ps, STDataType x) { assert(ps); if (ps->top == ps->capacity) { int newCapacity = ps->capacity == 0 ? 4 : (ps->capacity * 2); ps->a = (STDataType*)realloc(ps->a, sizeof(STDataType) * newCapacity); if (ps->a == NULL) { printf("realloc fail\n"); exit(-1); } ps->capacity = newCapacity; }
ps->a[ps->top] = x; ps->top++; }
void StackPop(ST* ps) { assert(ps); assert(ps->top > 0); --ps->top; }
int StackSize(ST* ps) { assert(ps); return ps->top; }
bool StackEmpty(ST* ps) { assert(ps);
return (ps->top == 0); }
STDataType StackTop(ST* ps) { assert(ps); assert(ps->top > 0); return ps->a[ps->top - 1]; }
void Destroy(ST* ps) { assert(ps); free(ps->a); ps->top = ps->capacity = 0; }
|
🎈结语
都看到这里了点个赞呗
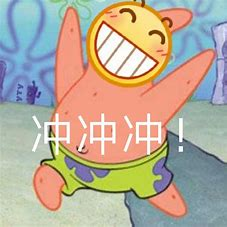
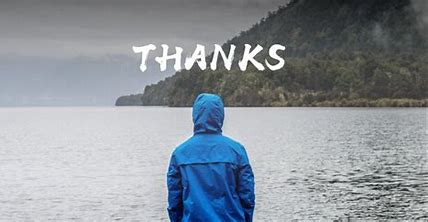